FOSDEM 2012 in Brussels
2012-02-26
Really need to add a blog entry about this - I was there a few weeks ago. Was coding some Wesnoth related stuff: units.wesnoth.org Also not directly related, I made a Pacman clone while bored: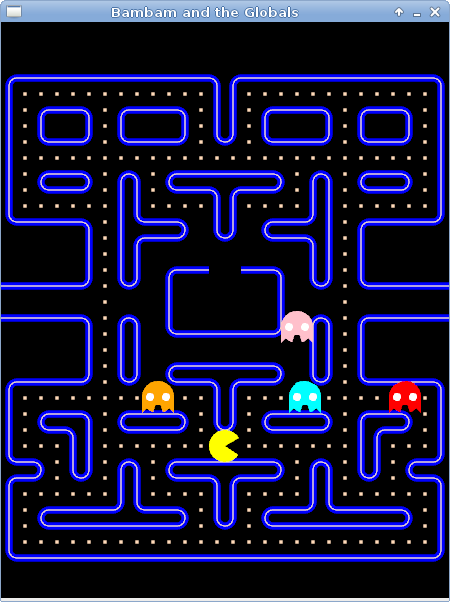
It uses no external datafiles, just plain Allegro 5 primitives:
#include <allegro5/allegro.h> #include <allegro5/allegro_primitives.h> #include <allegro5/allegro_color.h> #include <math.h>
ALLEGRO_DISPLAY *d; ALLEGRO_EVENT_QUEUE *q; ALLEGRO_EVENT e; ALLEGRO_TIMER *t; ALLEGRO_COLOR c; bool k[ALLEGRO_KEY_MAX]; int w = 30; int h = 38; int s = 16; int a, b, g; double p = ALLEGRO_PI, f; bool r; int x, y, px, py, kx, ky, sx, sy; int gx[4], gy[4], dx[4], dy[4]; char n[] = " " " " " " " " " ############################ " " #............##............# " " #.####.#####.##.#####.####.# " " #.# #.# #.##.# #.# #.# " " #.####.#####.##.#####.####.# " " #..........................# " " #.####.##.########.##.####.# " " #.####.##.########.##.####.# " " #......##....##....##......# " " ######.##### ## #####.###### " " #.##### ## #####.# " " #.## [] ##.# " " #.## ### ### ##.# " " ######.## #~~~~~~# ##.###### " " . #[][][]# . " " ######.## #~~~~~~# ##.###### " " #.## ######## ##.# " " #.## ##.# " " #.## ######## ##.# " " ######.## ######## ##.###### " " #............##............# " " #.####.#####.##.#####.####.# " " #.####.#####.##.#####.####.# " " #...##.......().......##...# " " ###.##.##.########.##.##.### " " ###.##.##.########.##.##.### " " #......##....##....##......# " " #.##########.##.##########.# " " #.##########.##.##########.# " " #..........................# " " ############################ " " " " " " "; char *m; #define P(x) (((x) - 1) * s) #define Q(x) (1 + (x) / s) #define M(x, y) m[(y) * w + (x)]
int main() { srand(time(NULL)); al_init(); al_install_keyboard(); al_init_primitives_addon(); al_set_new_display_option(ALLEGRO_SAMPLE_BUFFERS, 1, ALLEGRO_SUGGEST); al_set_new_display_option(ALLEGRO_SAMPLES, 8, ALLEGRO_SUGGEST); d = al_create_display((w - 2) * s, (h - 2) * s); al_set_window_title(d, "Bambam and the Globals"); q = al_create_event_queue(); t = al_create_timer(1.0 / 60); al_register_event_source(q, al_get_keyboard_event_source()); al_register_event_source(q, al_get_timer_event_source(t)); al_register_event_source(q, al_get_display_event_source(d)); al_start_timer(t); m = malloc(strlen(n) + 1); restart: strcpy(m, n); b = 0; y = 1; starty: x = 1; startx: if (M(x, y) != '(') goto noparenthesis; px = P(x) + s; py = P(y) + s / 2; noparenthesis: if (M(x, y) != '[') goto nobracket; gx[b] = P(x) + s; gy[b] = P(y) + s / 2; if (b == 0) goto notfirst; sx = gx[0]; sy = gy[0]; notfirst: b++; nobracket: x++; if (x < w - 1) goto startx; y++; if (y < h - 1) goto starty; mainloop: if (!r || !al_is_event_queue_empty(q)) goto dontdraw; al_clear_to_color(al_color_name("black")); b = 7; loopdrawhighlight: c = al_color_name("blue"); if (b == 1) c = al_color_name("wheat"); y = 1; loopdrawy: x = 1; loopdrawx: if (M(x, y) != '.') goto nodot; al_draw_filled_rectangle( P(x) + s * 0.4, P(y) + s * 0.4, P(x) + s * 0.6, P(y) + s * 0.6, al_color_name("peachpuff")); nodot: if (M(x, y) != '#') goto nohash; a = 1 * (M(x + 0, y - 1) == '#') + 2 * (M(x + 1, y - 1) == '#') + 4 * (M(x + 1, y + 0) == '#') + 8 * (M(x + 1, y + 1) == '#') + 16 * (M(x + 0, y + 1) == '#') + 32 * (M(x - 1, y + 1) == '#') + 64 * (M(x - 1, y + 0) == '#') + 128 * (M(x - 1, y - 1) == '#');
if (a == 64) al_draw_line(P(x), P(y) + s / 2, P(x) + s, P(y) + s / 2, c, b); else if (a == 4) al_draw_line(P(x), P(y) + s / 2, P(x) + s, P(y) + s / 2, c, b);
else if ((a & 85) == 5) al_draw_arc(P(x) + s, P(y), s / 2, p / 2, p / 2, c, b); else if ((a & 85) == 20) al_draw_arc(P(x) + s, P(y) + s, s / 2, p, p / 2, c, b); else if ((a & 85) == 65) al_draw_arc(P(x), P(y), s / 2, 0, p / 2, c, b); else if ((a & 85) == 80) al_draw_arc(P(x), P(y) + s, s / 2, p * 1.5, p / 2, c, b);
else if ((a & 7) == 5) al_draw_arc(P(x) + s, P(y), s / 2, p / 2, p / 2, c, b); else if ((a & 28) == 20) al_draw_arc(P(x) + s, P(y) + s, s / 2, p, p / 2, c, b); else if ((a & 193) == 65) al_draw_arc(P(x), P(y), s / 2, 0, p / 2, c, b); else if ((a & 112) == 80) al_draw_arc(P(x), P(y) + s, s / 2, p * 1.5, p / 2, c, b);
else if ((a & 17) == 17) al_draw_line(P(x) + s / 2, P(y), P(x) + s / 2, P(y) + s, c, b);
else if ((a & 68) == 68) al_draw_line(P(x), P(y) + s / 2, P(x) + s, P(y) + s / 2, c, b); nohash: x++; if (x < w - 1) goto loopdrawx; y++; if (y < h - 1) goto loopdrawy; b -= 6; if (b > 0) goto loopdrawhighlight;
b = 0; looptwice: al_draw_filled_pieslice(px + b * (w - 2) * s, py, s, f + p * 0.25 * fabs(sin(2 * p * (g % 60) / 60.0)), p * 2 - p * 0.5 * fabs(sin(2 * p * (g % 60) / 60.0)), al_color_name("yellow")); b++; if (b < 2) goto looptwice; b = 0; loopdrawghosts:
if (b == 0) c = al_color_name("red"); if (b == 1) c = al_color_name("cyan"); if (b == 2) c = al_color_name("pink"); if (b == 3) c = al_color_name("orange"); x = gx[b]; y = gy[b]; al_draw_filled_pieslice(x, y, s, p * 0.6, p * 1.8, c); al_draw_filled_triangle(x, y, x - s, y, x - s, y + s, c); al_draw_filled_triangle(x, y, x + s, y, x + s, y + s, c); al_draw_filled_rectangle(x - s * 0.5, y, x + s * 0.5, y + s * 0.5, c); al_draw_filled_circle(x - s * 0.5, y, s * 0.25, al_color_name("white")); al_draw_filled_circle(x + s * 0.5, y, s * 0.25, al_color_name("white"));
b++; if (b < 4) goto loopdrawghosts;
al_flip_display(); g++; r = false;
dontdraw: al_wait_for_event(q, &e); if (e.type == ALLEGRO_EVENT_DISPLAY_CLOSE) goto done; if (e.type != ALLEGRO_EVENT_TIMER) goto nottimer; if (k[ALLEGRO_KEY_ESCAPE]) goto done; if (k[ALLEGRO_KEY_LEFT]) {kx = -1; ky = 0; f = p;} if (k[ALLEGRO_KEY_RIGHT]) {kx = 1; ky = 0; f = 0;} if (k[ALLEGRO_KEY_UP]) {kx = 0; ky = -1; f = p * 1.5;} if (k[ALLEGRO_KEY_DOWN]) {kx = 0; ky = 1; f = p * 0.5;}
if (M(Q(px + kx * s / 2), Q(py + ky * s / 2)) == '#') goto cango; px += kx; py += ky; if (px < -s) px += (w - 2) * s; if (px > (w - 3) * s) px -= (w - 2) * s; if (M(Q(px + kx * s), Q(py + ky * s)) == '.') M(Q(px + kx * s), Q(py + ky * s)) = ' '; cango:
b = 0; loopghosts: x = gx[b] - px; y = gy[b] - py; if (x * x + y * y < s * s * 2 * 2) goto restart; if (dx[b] == 0 && dy[b] == 0) dx[b] = (rand() % 2) * 2 - 1;
if (M(Q(gx[b]), Q(gy[b])) != '~') goto noteleport; gx[b] = sx; gy[b] = sy; dy[b] = 0; noteleport:
if (M(Q(gx[b] + dx[b] * s / 2), Q(gy[b] + dy[b] * s / 2)) == '#') goto ghostwall; gx[b] += dx[b]; gy[b] += dy[b]; goto ghostmoved; ghostwall: if (dx[b] == 0) goto nodx; dx[b] = 0; dy[b] = (rand() % 2) * 2 - 1; goto ghostmoved; nodx: dy[b] = 0; dx[b] = (rand() % 2) * 2 - 1; ghostmoved: b++; if (b < 4) goto loopghosts;
r = true; goto mainloop; nottimer: if (e.type == ALLEGRO_EVENT_KEY_DOWN) k[e.keyboard.keycode] = true; if (e.type == ALLEGRO_EVENT_KEY_UP) k[e.keyboard.keycode] = false;
goto mainloop; done: free(m); al_uninstall_system(); }